Vex Serial Nodejs, Protocol, Packets, Irregular, Connection & More
Introduction
In our latest summer update, we’re excited to introduce a redesigned toolchain with the new cargo-v5 replacing cargo-pros. Unlike its predecessor, cargo-v5 no longer relies on pros-cli for uploading or terminal interactions. Instead, it integrates with the newly developed vex-v5-serial library, which is a complete reworking of the V5 Serial Protocol, fully implemented in Rust. This library supports various connection types, including wired, controller, and Bluetooth (btle), for enhanced flexibility.
Reengineering the Brain’s serial protocol was a significant undertaking, made possible with valuable resources like vexrs-serial (initially the basis for vex-v5-serial before it was entirely rewritten), v5-serial-protocol, and pros-cli. We are incredibly grateful to these open-source projects for their foundational contributions.
In this post, we’ll dive into the specifics of the V5 Serial Protocol and the technical aspects of vex-v5-serial’s development. This guide aims to be accessible to readers with foundational systems programming knowledge, even those unfamiliar with VEX robotics. In this article we;ll read about Vex Serial Nodejs.
The Serial Protocol
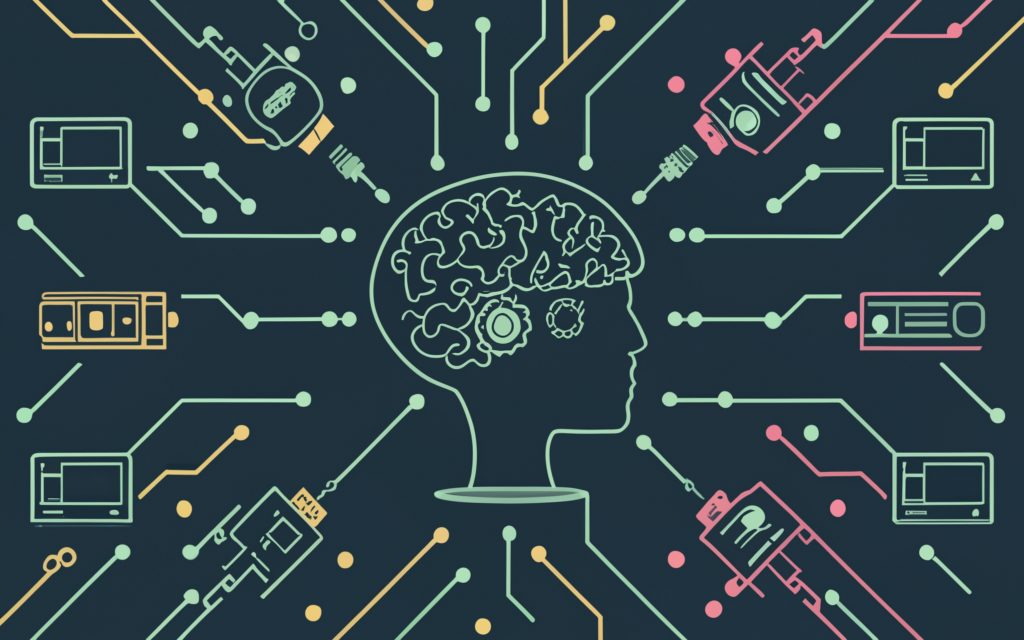
The V5 Serial Protocol is essentially a communication bridge between your computer and the V5 Brain, enabling tools like pros-cli and VEXcode to upload programs, view terminal outputs, and more. While it’s called a serial protocol, it supports both USB and Bluetooth connections, providing versatile ways to interface with the V5 Brain.
This protocol functions through two main types of binary packets: Command (sent from the computer to the Brain) and Response (sent from the Brain to the computer). Every Command packet initiates a corresponding Response. The packets are further categorized into CDC and CDC2 types (CDC stands for Communications Device Class). Command packets are identifiable by a specific header ([0xC9, 0x36, 0xB8, 0x47]) and an ID byte, while Response packets have a distinct host-bound header ([0xAA, 0x55]) with a matching ID. The content within these packets varies depending on the category and packet type, with both CDC and CDC2 packets capable of carrying an optional payload for additional data.
CDC2 Packets
CDC2 packets form the bulk of the V5 Serial Protocol’s data exchanges, designed with several unique features to enhance reliability and functionality. Unlike simpler CDC packets, CDC2 packets incorporate extended IDs and CRC16 checksums, providing added integrity and control.
When a CDC2 packet is sent to a device, it contains several key components:
The device-bound packet header signals the start of the packet.
A one-byte ID typically shared across most CDC2 packet types.
A unique extended ID for each type of CDC2 packet, offering more specific identification.
A VarU16 field that indicates the payload size, followed by the payload bytes themselves.
Finally, a CRC16 checksum is included to verify the accuracy and completeness of the entire packet.
These design elements make CDC2 packets more robust by ensuring that each packet can be verified and processed correctly, adding a layer of reliability essential for complex operations. This structure also allows CDC2 packets to support a wide range of commands, making them suitable for diverse and flexible device communications.
Irregular Packets
The ReadFileReplyPacket is a unique packet in the V5 Serial Protocol that doesn’t align perfectly with either the CDC or CDC2 packet types due to its flexible structure. When an error occurs, the packet includes specific fields to signal failure, but if the read operation is successful, the packet carries different data, making it versatile yet unconventional within the protocol.
On failure, the ReadFileReplyPacket includes:
An ID and extended ID to identify the packet type.
An ACK code signaling the error or issue.
A CRC16 checksum to verify the packet’s integrity.
In contrast, a successful read contains:
The ID and extended ID for identification.
The address of the memory location that was accessed.
The data that was retrieved from the read operation.
A CRC16 checksum for validation.
Because it doesn’t always include an ACK code and utilizes an extended ID, this packet type is set apart from typical CDC or CDC2 packets, reflecting a specialized design tailored to the protocol’s needs for flexible data retrieval.
Connection to the Brain
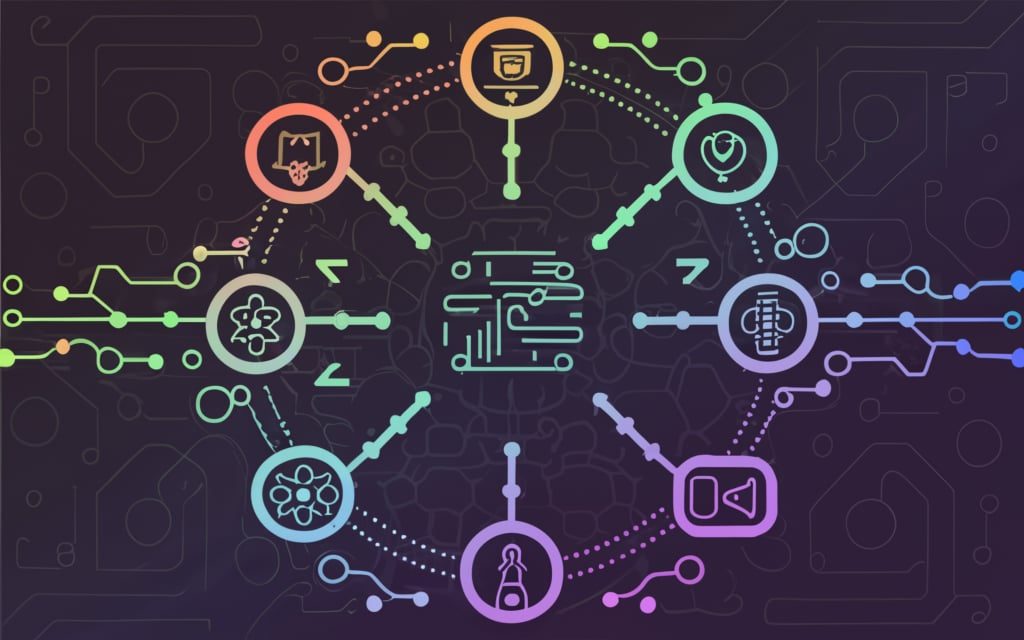
There are three primary methods to establish a connection between a host machine and a V5 Brain, each offering different advantages depending on the setup.
Direct Wired Connection: This method involves connecting your computer directly to the V5 Brain via a USB cable, providing the fastest communication speed. The connection utilizes two distinct serial ports: the system port, which facilitates communication with the Brain through the serial protocol, and the user port, which outputs user program data (often referred to as the debug terminal). These ports are crucial for effective communication with the Brain.
Controller Connection: In this setup, a USB connection links your controller to the V5 Brain via VEXnet, Bluetooth, or a physical cable. This connection is similar to a direct wired connection but only uses a single serial port, often referred to as the controller port. This port serves the same purpose as the system port in direct connections, but the program output is managed via a UserFifoPacket, which retrieves the incoming standard output buffer.
Bluetooth Connection: This method uses Bluetooth to connect directly to the V5 Radio, which then communicates with the Brain. While similar to a controller connection, it operates over Bluetooth and requires the radio mode to be set to Bluetooth with data enabled in the Brain settings. However, this is considered the least secure connection method, so it’s recommended to disable data when not in use for added security.
How vex-v5-serial Works
The vex-v5-serial challenge is designed with a modular structure, where the code is prepared into numerous wonderful components, every dealing with a selected characteristic. This method not best complements the general nice of the code but additionally allows for greater flexibility and customization. By breaking the assignment into smaller, attainable sections, it turns into less difficult to preserve and update person elements without affecting the entire machine.
One of the key benefits of this modular layout is that it supports the implementation of numerous capabilities that interact with more than one sections of the code. For example, the connection feature is answerable for dealing with the connection good judgment, and it may be toggled on or off as needed. This lets in for seamless control of the connection method, whether via USB, Bluetooth, or different interfaces, with out interfering with other gadget additives.
By organizing the challenge on this manner, vex-v5-serial becomes now not only more green however also less complicated to extend or regulate inside the destiny, making it an improved solution for developers running with VEX Robotics systems. This established layout contributes to the overall reliability and scalability of the mission, making sure that future updates may be carried out with minimum disruption.
Packets
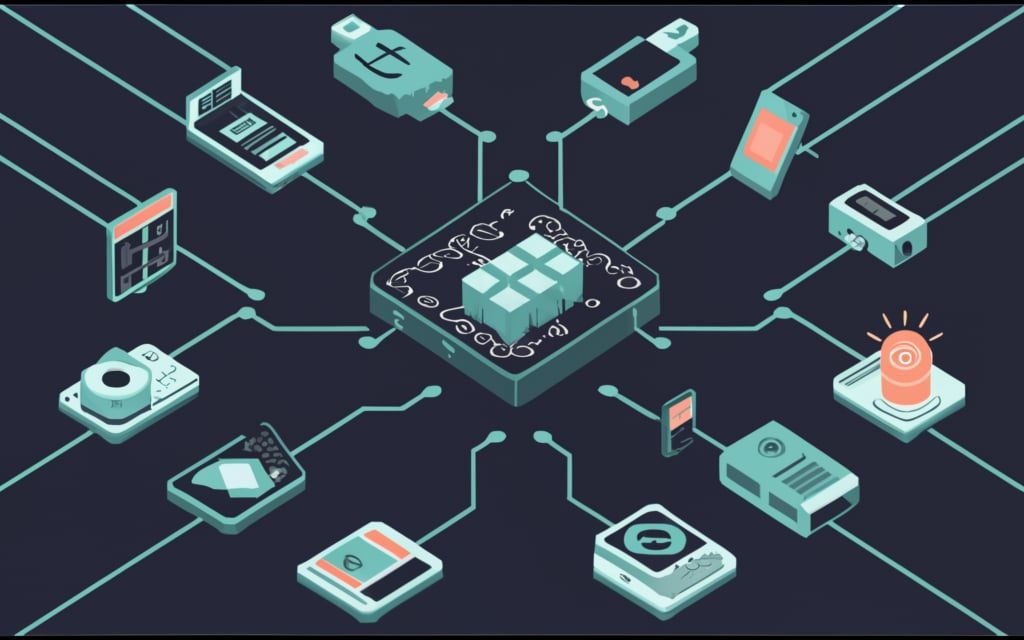
The vex-v5-serial project handles a diverse set of around 100 unique packet types, both Brain-bound and host-bound. To efficiently manage this large number, these packets are organized within submodules of a broader packets module, ensuring a clean and structured approach to handling each type.
The project defines four primary packet types that serve as the foundation for encoding and decoding within the protocol, excluding the ReadFileReplyPacket. These core packet types include: CdcReplyPacket, Cdc2ReplyPacket, CdcCommandPacket, and Cdc2CommandPacket.
Each of these packet types is designed with parameters to specify crucial details like the packet ID, extended ID (if applicable), and the payload type. The payload type is represented as () when the packet does not carry any payload, ensuring clear distinction between packet types that contain data and those that do not. This parameterized structure simplifies the creation of new packet types, enabling efficient and flexible handling of various communication needs across the protocol.
This design makes the system scalable and adaptable, as new packet types can be easily integrated while maintaining the integrity and clarity of the entire communication framework.
Encoding and Decoding
In the vex-v5-serial project, two key traits are utilized to handle the encoding and decoding of packets: Encode and Decode. These traits facilitate the seamless transformation between command packets and their corresponding response packets.
The Encode trait takes a Command packet and converts it into a byte vector, ready for transmission to the Brain. On the other hand, the Decode trait processes the byte vector it receives and reconstructs it into a Response packet. For those with a keen interest in parsing, Decode functions as an LL(1) parser, which is designed to efficiently process simple data structures.
While Decode works well for most scenarios, it does have limitations, particularly when dealing with arrays or strings that aren’t null-terminated. To address this, a third trait, SizedDecode, can be employed, though it’s used less frequently. Despite these challenges, the simplicity of the Decode trait allows developers to quickly build efficient parsers that are both powerful and easy to use. This approach ensures high performance while maintaining an ergonomic and user-friendly interface for packet processing. Here’s an example based on real code that demonstrates how this parsing process works in practice.
FACT:
- vex-v5-serial Library: This library is a complete rewrite of the V5 Serial Protocol, implemented in Rust. It replaces cargo-pros with cargo-v5, integrating connection types like USB, controller, and Bluetooth (BTLE).
- V5 Serial Protocol: It enables communication between a computer and the V5 Brain, supporting both USB and Bluetooth. It involves Command and Response packets with CDC and CDC2 types, which contain various headers, IDs, and payloads.
- CDC2 Packets: These are designed with extended IDs and CRC16 checksums for added reliability. They contain a packet header, ID, extended ID, payload size, and a checksum for verification.
- ReadFileReplyPacket: A unique packet type that can either signal an error or return data from a successful read. It’s distinct due to its flexible structure.
- Connection Methods:
- Wired: Fastest communication via USB using system and user ports.
- Controller: Uses USB, VEXnet, or Bluetooth to connect via a controller port.
- Bluetooth: Connects via Bluetooth to the V5 Radio, but considered less secure.
- Modular Structure: The vex-v5-serial project is modular, making it easier to maintain and expand. The connection logic is flexible, toggling between interfaces like USB or Bluetooth.
- Packet Types: About 100 packet types are managed in submodules. Key packet types include CdcReplyPacket, Cdc2ReplyPacket, CdcCommandPacket, and Cdc2CommandPacket, with flexible parameters for ID and payloads.
- Encoding and Decoding:
- Encode: Converts Command packets into byte vectors for transmission.
- Decode: Reconstructs Response packets from byte vectors, using an LL(1) parser for efficiency. A third trait, SizedDecode, addresses specific limitations with non-null-terminated strings and arrays.
These points summarize the technical facts and core details of the vex-v5-serial library and the V5 Serial Protocol.
FAQs:
1. What is the V5 Serial Protocol?
The V5 Serial Protocol is a communication bridge between your computer and the V5 Brain, enabling tools like pros-cli and VEXcode to upload programs, view terminal outputs, and more. It supports both USB and Bluetooth connections, allowing versatile interaction with the V5 Brain.
2. What are CDC and CDC2 packets?
CDC and CDC2 are types of binary packets used in the V5 Serial Protocol. CDC packets are simpler, while CDC2 packets are more robust, incorporating extended IDs and CRC16 checksums for added integrity and control in communication.
3. What is the ReadFileReplyPacket?
The ReadFileReplyPacket is a unique packet that doesn’t strictly follow the CDC or CDC2 format. It can either signal an error or return data from a successful read operation, depending on its structure.
4. How do I establish a connection with the V5 Brain?
There are three primary connection methods:
- Wired: Fastest communication via USB with two distinct serial ports.
- Controller: Connects through USB, VEXnet, or Bluetooth via a controller port.
- Bluetooth: Uses Bluetooth to connect to the V5 Radio, though it is considered less secure.
5. What is the modular structure of the vex-v5-serial project?
The vex-v5-serial project is designed with a modular structure, allowing flexibility and ease of maintenance. It separates the functionality into smaller components, making it easier to manage and extend in the future.
6. How are packets handled in the vex-v5-serial library?
The vex-v5-serial library organizes packets into submodules and defines core packet types like CdcReplyPacket, Cdc2ReplyPacket, CdcCommandPacket, and Cdc2CommandPacket. These packets are parameterized to specify details like IDs and payload types, allowing efficient handling and encoding/decoding.
7. How does the encoding and decoding process work?
The Encode trait converts Command packets into byte vectors for transmission, while the Decode trait processes byte vectors into Response packets. An LL(1) parser is used for efficient parsing, and a third trait, SizedDecode, helps with non-null-terminated strings and arrays.
8. What connection types does vex-v5-serial support?
vex-v5-serial supports USB, controller (VEXnet, Bluetooth, or physical cable), and Bluetooth (via V5 Radio) connections for communicating with the V5 Brain.
Summary:
The article introduces the vex-v5-serial library, a complete rewrite of the V5 Serial Protocol, implemented in Rust. It replaces the previous cargo-pros tool with cargo-v5, offering enhanced flexibility and support for various connection types, including USB, controller (VEXnet, Bluetooth), and Bluetooth (via V5 Radio).
The V5 Serial Protocol enables communication between a computer and the V5 Brain through Command and Response packets, categorized into CDC and CDC2 types. CDC2 packets offer added reliability with extended IDs and CRC16 checksums. A unique packet, the ReadFileReplyPacket, can signal errors or return data, depending on the read operation’s success.
The vex-v5-serial project is modular, allowing easy maintenance and expansion. It handles around 100 packet types, structured into submodules for efficient processing. The library uses Encode and Decode traits to convert Command packets into byte vectors and reconstruct Response packets from byte vectors, respectively, with an LL(1) parser for efficiency. Additionally, it supports the SizedDecode trait for more complex data handling.
Read More Information About Technology visit Discover Paradox